Using External APIs in RoR
Audience1st uses figaro
to manage secrets. As mentioned in the Get Started with Legacy Project. And it uses Stripe
to deal with online payments.
Figaro
figaro
will generate a git-ignored file config/application.yml
for secrets management. In Audience1st
, it looks like this:
test:
session_secret: "30 or more random characters string"
stripe_key: "stripe public (publishable) key"
stripe_secret: "stripe private API key"
# include at most one of the following two lines - not both:
email_integration: "MailChimp" # if you use MailChimp, include this line verbatim, else omit
email_integration: "ConstantContact" # if you use CC, include this line verbatime, else omit
# if you included one of the two Email Integration choices:
mailchimp_key: "optional: if you use Mailchimp, API key; otherwise omit this entry"
constant_contact_username: "Username for CC login, if using CC"
constant_contact_password: "password for CC login, if using CC"
constant_contact_key: "CC publishable part of API key"
constant_contact_secret: "CC secret part of API key
You can use ENV['stripe_key']
or Figaro.env.stripe_key
, which totally depends on personal preference, to access the secrets you set in config/application.yml
. Using a tool to manage secrets is a very good practice in terms of separating dependencies.
Stripe
You should never include your API keys in plaintext as is showed in the API documentations of Stripe
, because they do this for readability. You can get your api_key for free by creating an account at stripe.com
Here’s a typical charge action using stripe
, it will return a JSON
object:
require "stripe"
Stripe.api_key = "wublubdabuda"
Stripe::Charge.create({
# 2000 means $20.00, the unit is *cent*
:amount => 2000,
:currency => "usd",
:source => "tok_mastercard", # obtained with Stripe.js
:description => "Charge for jackwan@example.com"
}, {
:idempotency_key => "whateverulike"
})
NOTE: Credit card information is sent directly to Stripe, ensuring sensitive data never hits your servers, all you get is a token.
NOTE FURTHER: Genuine card information cannot be used in test mode. Instead, use any of the following test card numbers, a valid expiration date in the future, and any random CVC number, to create a successful payment.
Number | Brand |
---|---|
4242424242424242 | Visa |
4000056655665556 | Visa (debit) |
5555555555554444 | Mastercard |
5200828282828210 | Mastercard (debit) |
5105105105105100 | Mastercard (prepaid) |
378282246310005 | American Express |
371449635398431 | American Express |
6011111111111117 | Discover |
6011000990139424 | Discover |
30569309025904 | Diners Club |
38520000023237 | Diners Club |
3530111333300000 | JCB |
To better understand Stripe
’s working mechanism, check this out:
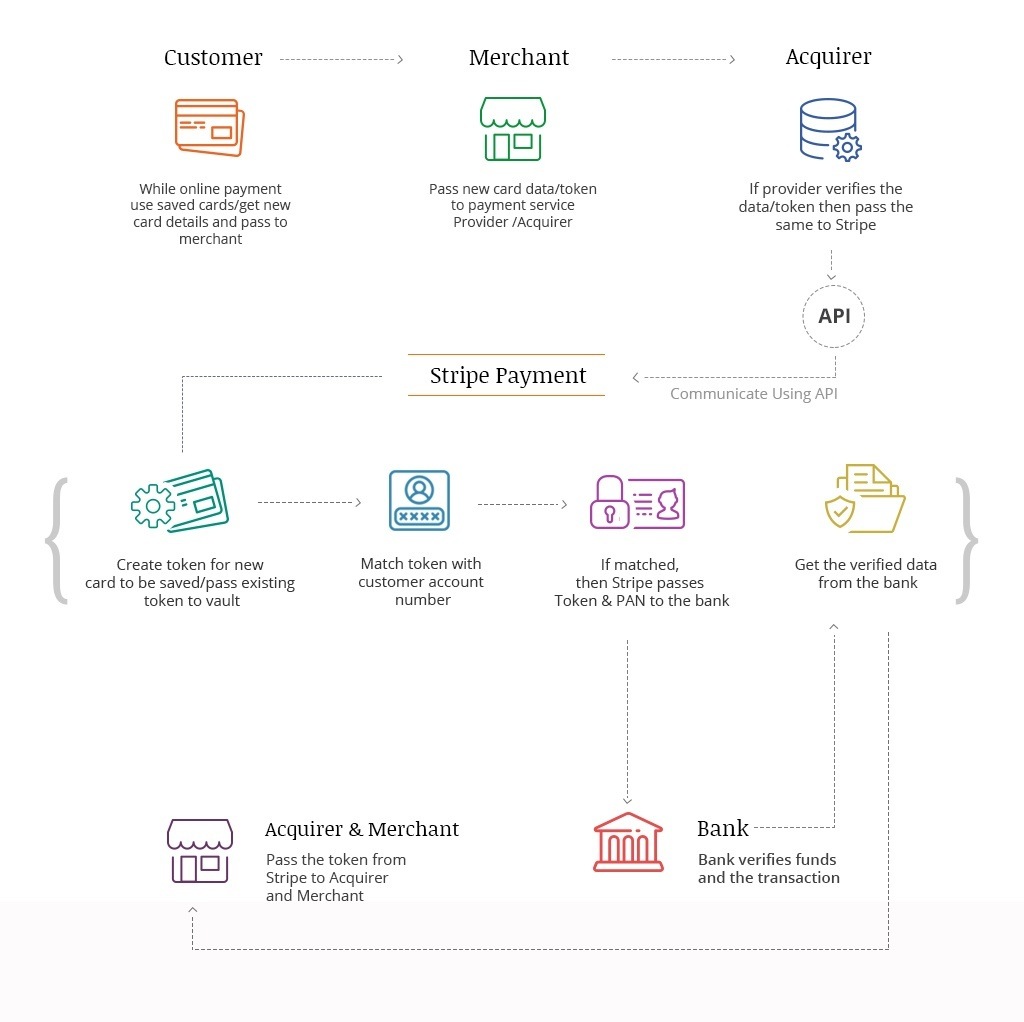